Welcome to Gamespublisher.com, your go-to source for everything related to game development and publishing. In today’s rapidly evolving gaming industry, understanding Java games programming is essential for aspiring and seasoned developers alike.
Java, a versatile and powerful programming language, has played a significant role in the development of engaging and immersive games across various platforms.
Java’s rise as a programming language is nothing short of remarkable. From its inception by Sun Microsystems to its current status as a staple in the gaming industry, Java has consistently provided developers with a robust and flexible environment for creating games.
This comprehensive guide will delve into the intricacies of Java game development, offering insights, tutorials, and best practices to help you excel in this exciting field.
1. Overview of Java Games Programming
History and Evolution of Java in Gaming
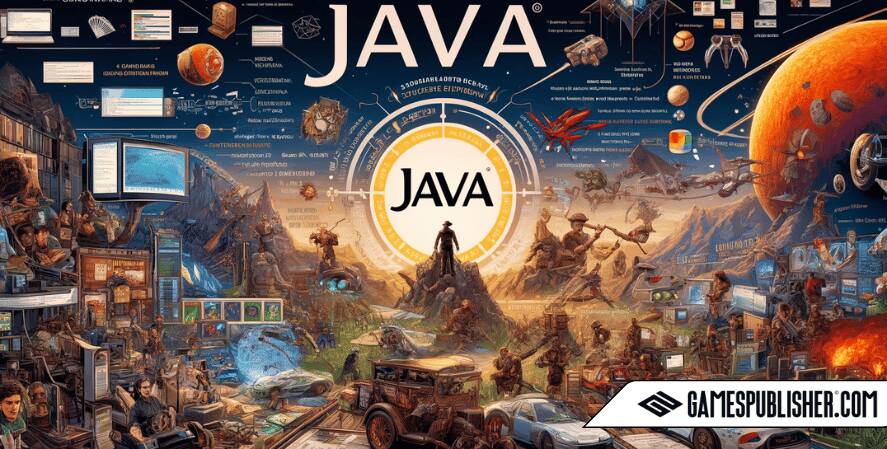
Java was developed by Sun Microsystems in the mid-1990s, with the initial goal of creating a language that could be implemented across a wide range of devices. Its “write once, run anywhere” philosophy made it an attractive option for developers.
Over the years, Java has evolved significantly, incorporating new features and improvements that have enhanced its utility in various domains, including gaming.
Key milestones in Java’s journey within the gaming industry include the introduction of Java 2D and JavaFX, which provided advanced graphical capabilities.
These tools allowed developers to create more sophisticated and visually appealing games. The rise of mobile gaming further cemented Java’s position, as it became the language of choice for many Android game developers.
Why Choose Java for Game Development?
Java offers several advantages for game development, making it a popular choice among developers:
- Platform Independence: Java’s ability to run on any device with a Java Virtual Machine (JVM) ensures that games can reach a wide audience without the need for extensive modifications.
- Ease of Use: Java’s syntax is relatively simple and easy to learn, which accelerates the development process and makes it accessible to beginners.
- Large Developer Community: Java boasts a vast and active developer community, providing ample resources, libraries, and frameworks that can be leveraged to enhance game development.
- Robust Libraries and Frameworks: Java’s extensive libraries, such as Java 2D, JavaFX, and popular game frameworks like LibGDX and jMonkeyEngine, offer powerful tools for creating complex games.
When compared to other popular game programming languages like C++ and Python, Java stands out for its balance of performance and ease of use. While C++ offers superior performance and control over hardware resources, it has a steeper learning curve.
Python, on the other hand, is easier to learn but may not provide the same level of performance for resource-intensive games. Java strikes a middle ground, offering both efficiency and ease of development.
2. Getting Started with Java for Game Development
Setting Up the Development Environment
To begin your journey in Java game development, you’ll need to set up the appropriate development environment. Here’s a step-by-step guide:
- Install Java Development Kit (JDK): Download and install the latest version of JDK from the official Oracle website.
- Select an Integrated Development Environment (IDE): Commonly used IDEs for Java development are Eclipse, IntelliJ IDEA, and NetBeans. These tools offer powerful features that streamline the coding process.
- Set Up Project Structure: Create a new project in your chosen IDE, and configure the necessary libraries and dependencies required for game development. Ensure that your project is well-organized to facilitate easy maintenance and scalability.
Basic Concepts and Syntax of Java
Understanding the basic concepts and syntax of Java is crucial for game development. Here are some fundamental principles:
- Classes and Objects: Java is an object-oriented language, meaning it relies on the concept of classes and objects. Classes define the blueprint for objects, which are instances of classes.
- Methods and Functions: Methods are blocks of code that perform specific tasks. They are defined within classes and can be called upon to execute the code.
- Inheritance and Polymorphism: These are key principles of object-oriented programming that allow for the creation of flexible and reusable code.
Here’s a simple code example to illustrate these concepts:
javaCopy codepublic class Game {
public static void main(String[] args) {
Player player = new Player("John", 100);
player.displayPlayerInfo();
}
}
class Player {
String name;
int score;
Player(String name, int score) {
this.name = name;
this.score = score;
}
void displayPlayerInfo() {
System.out.println("Player Name: " + name);
System.out.println("Score: " + score);
}
}
3. Core Components of Java Game Development
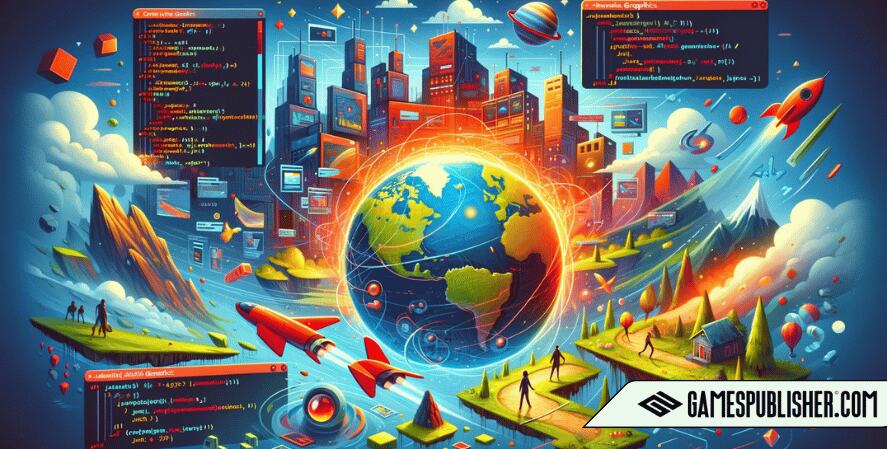
Graphics and Animation
Java offers robust graphics capabilities through Java 2D and JavaFX. These tools enable developers to create visually appealing games with smooth animations.
- Java 2D: This API provides advanced 2D graphics capabilities, allowing for the creation of shapes, text, and images.
- JavaFX: A more modern and versatile framework, JavaFX supports 2D and 3D graphics, along with animation and media playback.
Techniques for Creating and Managing Animations
Animations are crucial for creating dynamic and engaging games. Here’s a basic example of animation using JavaFX:
javaCopy codeimport javafx.animation.TranslateTransition;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.stage.Stage;
import javafx.util.Duration;
public class AnimationExample extends Application {
@Override
public void start(Stage stage) {
Rectangle rect = new Rectangle(50, 50, 100, 100);
rect.setFill(Color.BLUE);
TranslateTransition transition = new TranslateTransition();
transition.setDuration(Duration.seconds(2));
transition.setNode(rect);
transition.setByX(300);
transition.setAutoReverse(true);
transition.setCycleCount(TranslateTransition.INDEFINITE);
transition.play();
Scene scene = new Scene(new javafx.scene.Group(rect), 400, 400);
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Game Physics and Collision Detection
Implementing realistic physics and collision detection is essential for creating engaging games. Java provides various libraries and tools to facilitate this.
Basic Principles of Game Physics
Game physics involves simulating the physical behavior of objects. This includes gravity, friction, and collision responses. Libraries like JBox2D, a Java port of the popular Box2D physics engine, can be used to implement these features.
Collision Detection Example
Here’s a simple example of collision detection in a Java game:
javaCopy codepublic class CollisionDetection {
public static void main(String[] args) {
Rectangle rect1 = new Rectangle(0, 0, 50, 50);
Rectangle rect2 = new Rectangle(25, 25, 50, 50);
if (rect1.intersects(rect2.getBoundsInLocal())) {
System.out.println("Collision Detected!");
} else {
System.out.println("No Collision.");
}
}
}
class Rectangle {
int x, y, width, height;
Rectangle(int x, int y, int width, int height) {
this.x = x;
this.y = y;
this.width = width;
this.height = height;
}
java.awt.Rectangle getBoundsInLocal() {
return new java.awt.Rectangle(x, y, width, height);
}
}
User Input Handling
Capturing and responding to user inputs is a fundamental aspect of game development. Java provides straightforward ways to handle keyboard, mouse, and touch inputs.
Handling Keyboard Input
Here’s an example of handling keyboard input in a Java game:
javaCopy codeimport java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import javax.swing.JFrame;
public class KeyboardInputExample extends JFrame implements KeyListener {
public KeyboardInputExample() {
addKeyListener(this);
setSize(400, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
@Override
public void keyTyped(KeyEvent e) {}
@Override
public void keyPressed(KeyEvent e) {
if (e.getKeyCode() == KeyEvent.VK_LEFT) {
System.out.println("Left Arrow Key Pressed");
}
}
@Override
public void keyReleased(KeyEvent e) {}
public static void main(String[] args) {
new KeyboardInputExample();
}
}
4. Advanced Java Game Programming Techniques
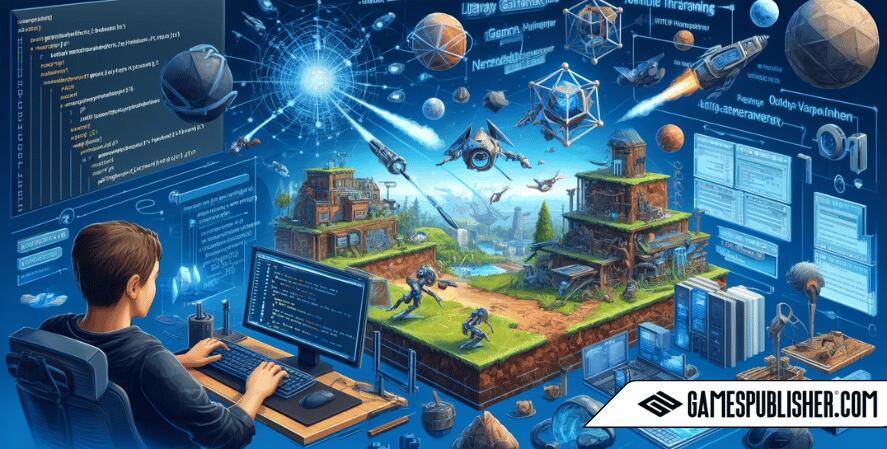
Using Libraries and Frameworks
Java offers several powerful libraries and frameworks for game development. Here are a few notable ones:
- LWJGL (Lightweight Java Game Library): Provides bindings to OpenGL, OpenAL, and OpenCL, facilitating high-performance games.
- LibGDX: A cross-platform framework that supports desktop, Android, iOS, and HTML5 games.
- jMonkeyEngine: A game engine designed for creating 3D games using modern techniques and practices.
Multithreading in Games
Multithreading is crucial for optimizing game performance, especially for handling complex tasks like rendering and physics calculations.
Implementing Multithreading
Here’s an example of using multithreading in a Java game:
javaCopy codepublic class GameLoop implements Runnable {
private boolean running = true;
@Override
public void run() {
while (running) {
update();
render();
try {
Thread.sleep(16); // Approx. 60 frames per second
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
private void update() {
// Update game state
}
private void render() {
// Render game graphics
}
public static void main(String[] args) {
GameLoop gameLoop = new GameLoop();
Thread thread = new Thread(gameLoop);
thread.start();
}
}
Networking and Multiplayer Games
Creating multiplayer games involves understanding networking principles and implementing client-server architecture.
Example of Creating a Multiplayer Game
Here’s a basic example of a server-client setup for a multiplayer game in Java:
Server:
javaCopy codeimport java.io.*;
import java.net.*;
public class GameServer {
public static void main(String[] args) throws IOException {
ServerSocket serverSocket = new ServerSocket(12345);
System.out.println("Server started...");
while (true) {
Socket socket = serverSocket.accept();
new Thread(new ClientHandler(socket)).start();
}
}
}
class ClientHandler implements Runnable {
private Socket socket;
ClientHandler(Socket socket) {
this.socket = socket;
}
@Override
public void run() {
try (BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
PrintWriter out = new PrintWriter(socket.getOutputStream(), true)) {
String message;
while ((message = in.readLine()) != null) {
System.out.println("Received: " + message);
out.println("Echo: " + message);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
Client:
javaCopy codeimport java.io.*;
import java.net.*;
public class GameClient {
public static void main(String[] args) {
try (Socket socket = new Socket("localhost", 12345);
BufferedReader in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
PrintWriter out = new PrintWriter(socket.getOutputStream(), true);
BufferedReader console = new BufferedReader(new InputStreamReader(System.in))) {
String userInput;
while ((userInput = console.readLine()) != null) {
out.println(userInput);
System.out.println("Server response: " + in.readLine());
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
5. Case Studies and Examples
Successful Java Games
Java has been the backbone of several successful games, with Minecraft being the most notable example. Developed by Mojang, Minecraft’s simplistic graphics and complex gameplay mechanics showcase Java’s capability in game development.
The game’s success is attributed to its flexibility, allowing extensive modding and cross-platform compatibility.
Step-by-Step Game Development Tutorial
To illustrate the process of game development in Java, let’s create a simple 2D game. Here’s a detailed tutorial:
- Setting Up: Start by creating a new Java project in your IDE and setting up the necessary libraries.
- Game Loop: Implement the main game loop to handle updates and rendering.
- Graphics: Use Java 2D to draw game elements on the screen.
- User Input: Capture user inputs to control game elements.
- Game Logic: Implement the core game logic, including collision detection and scoring.
Here’s a simple code example to get you started:
javaCopy codeimport javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyAdapter;
import java.awt.event.KeyEvent;
public class SimpleGame extends JPanel implements ActionListener {
private Timer timer;
private int x, y, velX, velY;
public SimpleGame() {
x = 0;
y = 0;
velX = 2;
velY = 2;
timer = new Timer(10, this);
timer.start();
addKeyListener(new KeyAdapter() {
@Override
public void keyPressed(KeyEvent e) {
int key = e.getKeyCode();
if (key == KeyEvent.VK_LEFT) {
velX = -2;
} else if (key == KeyEvent.VK_RIGHT) {
velX = 2;
} else if (key == KeyEvent.VK_UP) {
velY = -2;
} else if (key == KeyEvent.VK_DOWN) {
velY = 2;
}
}
});
setFocusable(true);
}
@Override
public void actionPerformed(ActionEvent e) {
x += velX;
y += velY;
repaint();
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
g.fillRect(x, y, 50, 50);
}
public static void main(String[] args) {
JFrame frame = new JFrame("Simple Game");
SimpleGame game = new SimpleGame();
frame.add(game);
frame.setSize(400, 400);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
6. Best Practices and Optimization
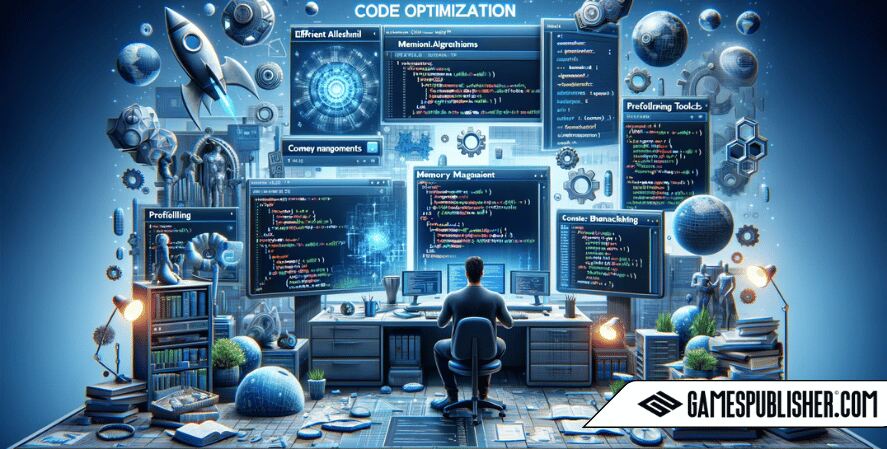
Code Optimization Techniques
Optimizing your code is crucial for ensuring smooth performance, especially in resource-intensive games. Here are some strategies:
- Efficient Algorithms: Use efficient algorithms and data structures to minimize computational overhead.
- Memory Management: Optimize memory usage by minimizing the creation of unnecessary objects and employing efficient data storage techniques.
- Profiling and Benchmarking: Regularly profile and benchmark your code to identify performance bottlenecks.
Testing and Debugging
Testing and debugging are critical components of game development. Best practices include:
- Unit Testing: Create unit tests to validate the functionality of specific components.
- Integration Testing: Ensure that different parts of the game work together seamlessly.
- Debugging Tools: Use debugging tools provided by your IDE to step through code and identify issues.
Conclusion
In this comprehensive guide, we’ve explored the world of Java game development, from setting up your development environment to advanced programming techniques and best practices.
Java’s platform independence, ease of use, and robust libraries make it an excellent choice for game developers. As the gaming industry continues to grow, so does the potential for Java in creating innovative and engaging games.
We encourage you to start experimenting with Java for your game development projects. The continuous growth of Java and its community promises a bright future for Java game developers. Happy coding!