Welcome to Gamespublisher.com, your go-to resource for aspiring and experienced game developers and publishers. Our mission is to support the creative minds behind the gaming industry’s most innovative titles by providing valuable insights, resources, and guidance.
In this comprehensive guide, we explore the role of Python in game development, highlighting its simplicity and flexibility, and demonstrating why it has become a popular choice for both beginners and seasoned developers.
Python’s importance in game development cannot be overstated. Python’s simplicity, clear syntax, and rich library ecosystem make it an ideal choice for game development.
This guide aims to provide a thorough understanding of Python’s capabilities in game development and offer practical advice for developing your games using this powerful language.
Overview of Python in Game Development
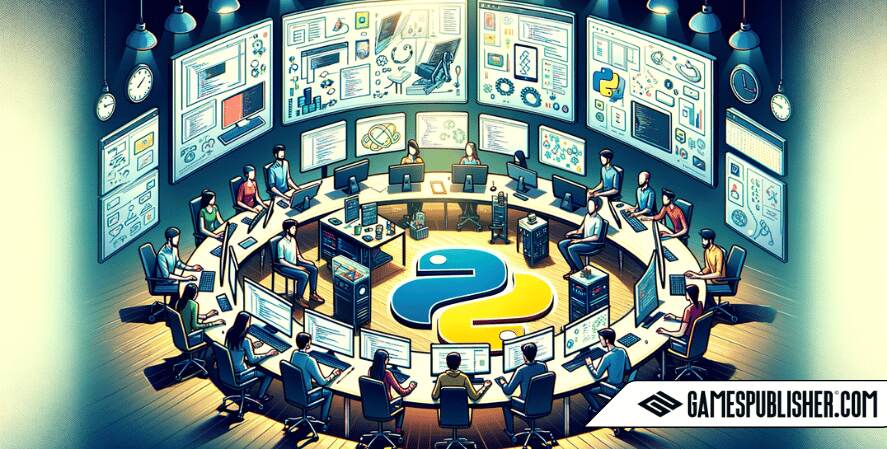
Brief History of Python and Its Rise in the Gaming Industry
Python was created in the late 1980s by Guido van Rossum and has since grown into one of the most popular programming languages in the world.
Initially designed with an emphasis on code readability and simplicity, Python has found applications in various fields, including web development, data science, artificial intelligence, and, notably, game development.
The gaming industry has seen a surge in Python’s popularity due to its ease of use and the powerful libraries available for game development. Python’s simplicity allows developers to quickly prototype and develop games, making it an attractive choice for game developers.
Advantages of Using Python for Game Development
Python offers numerous advantages for game development, including:
- Ease of Learning: Python’s straightforward syntax and readability make it an excellent choice for beginners.
- Readability: Python code is easy to read and understand, which helps in maintaining and debugging games.
- Extensive Libraries: Python boasts a rich ecosystem of libraries that simplify various aspects of game development, from graphics rendering to sound processing.
- Cross-Platform Compatibility: Python runs on multiple platforms, including Windows, macOS, and Linux, allowing developers to create games that work across different systems.
- Active Community: Python has a large and active community, providing a wealth of resources, tutorials, and support for game developers.
Comparison with Other Popular Game Development Languages
Python stands out among other game development languages such as C++, Java, and C#. While C++ is known for its performance and control, it has a steeper learning curve compared to Python.
Java offers portability and robustness but lacks the simplicity and readability of Python. C# is widely used with the Unity engine but is less versatile outside the Unity ecosystem.
Python strikes a balance between ease of use and functionality, making it a versatile choice for game development.
Essential Python Libraries for Game Development
Pygame
Introduction to Pygame and Its Core Functionalities
Pygame is a collection of Python modules that enable the creation of video games across different platforms. It includes computer graphics and sound libraries, which provide functionalities needed to create engaging games.
Key Features and How It Simplifies Game Development
- 2D Graphics Support: Pygame offers a wide range of functions for 2D graphics, making it easy to create visually appealing games.
- Sound Integration: The library supports sound effects and background music, enhancing the gaming experience.
- Input Handling: Pygame handles input from various devices, including keyboards, mice, and game controllers.
Here’s a basic example of a “Hello, World!” game using Pygame:
pythonCopy codeimport pygame
# Initialize Pygame
pygame.init()
# Set up display
screen = pygame.display.set_mode((640, 480))
pygame.display.set_caption("Hello, Pygame!")
# Main loop
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# Fill the screen with a color
screen.fill((0, 0, 255))
# Update the display
pygame.display.flip()
# Quit Pygame
pygame.quit()
Pyglet
Overview of Pyglet and Its Use for Multimedia and Game Programming
Pyglet is an alternative library used for creating games and multimedia applications with Python. It is known for its simplicity and performance, providing a windowing and multimedia library for OpenGL.
Comparison with Pygame and When to Use Pyglet
While Pygame is excellent for 2D game development, Pyglet offers better support for 3D graphics and OpenGL. Use Pyglet when you need advanced graphics features and performance.
Panda3D
Explanation of Panda3D and Its Capabilities for 3D Game Development
Panda3D is a game engine that supports 3D rendering and game development. It is developed by Disney and maintained by Carnegie Mellon University.
Benefits and Limitations of Using Panda3D
- Benefits: Panda3D offers powerful 3D rendering capabilities, a comprehensive set of tools, and a supportive community.
- Limitations: The learning curve can be steep for beginners, and it may require more system resources compared to 2D engines.
Kivy
Introduction to Kivy and Its Application in Developing Multi-Touch Applications
Kivy is an open-source Python library for developing multi-touch applications. It is suitable for mobile game development and supports various platforms, including Android and iOS.
How Kivy Can Be Used for Mobile Game Development
Kivy simplifies mobile game development by providing a framework that handles touch inputs, gestures, and device-specific functionalities.
Step-by-Step Guide to Creating a Basic Game in Python
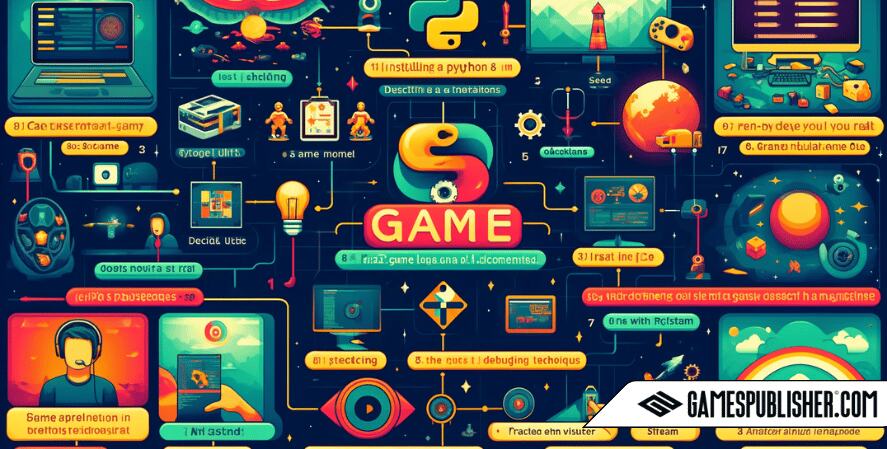
Installing Python and Necessary Libraries
First, download and install the latest version of Python from the official website. Next, install essential libraries like Pygame using pip:
bashCopy codepip install pygame
Setting Up an Integrated Development Environment (IDE)
Choose an IDE that suits your needs. Some widely used options are PyCharm, Visual Studio Code, and Sublime Text.
Designing Your Game
Conceptualizing the Game Idea
Start by brainstorming and defining the core concept of your game. What genre will it be? What is the main objective?
Creating a Game Design Document
Document your game’s mechanics, story, characters, and levels. This design document will guide you through the development process.
Coding the Game
Writing the Game Loop
The game loop is the core of your game, handling updates and rendering.
pythonCopy code# Game loop example
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# Update game state
# Render game frame
pygame.display.flip()
Implementing Game Mechanics (Controls, Score, Levels)
Define the controls and implement the game mechanics such as player movement, scoring, and level progression.
Adding Graphics and Sound
Integrate graphics and sound assets to enhance the gaming experience. Pygame provides functions to load and display images and play sounds.
Testing and Debugging
Common Debugging Techniques
Use print statements and debugging tools provided by your IDE to troubleshoot issues.
How to Test Your Game for Different Scenarios
Playtest your game to identify and fix bugs. Consider beta testing with a small group of users to get feedback.
Packaging and Distribution
Packaging Your Game for Different Platforms
Use tools like PyInstaller to package your game into executable files for different platforms.
bashCopy codepip install pyinstaller
pyinstaller --onefile your_game.py
Distribution Channels and Marketing Tips
Distribute your game through platforms like Steam, Itch.io, or the Google Play Store. Promote your game through social media, gaming forums, and by reaching out to influencers.
Advanced Topics in Python Game Development
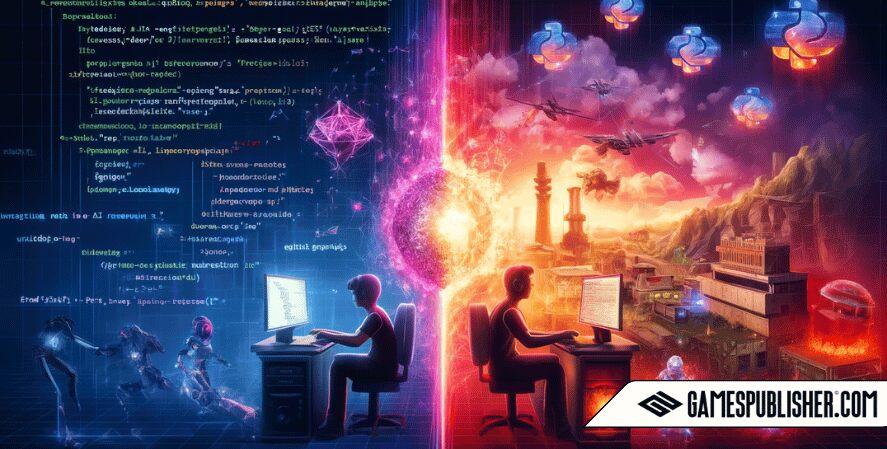
Optimizing Performance
Techniques to Improve Game Performance
- Efficient Coding Practices: Optimize your code to reduce unnecessary computations.
- Memory Management: Manage resources effectively to prevent memory leaks.
Integrating AI in Games
Basic AI Concepts and Their Application in Games
Implement AI for non-player characters (NPCs) to enhance gameplay. Use algorithms like A* for pathfinding and decision trees for NPC behavior.
Example of Implementing a Simple AI Opponent
pythonCopy code# Simple AI example
if player.position.x > npc.position.x:
npc.move_right()
elif player.position.x < npc.position.x:
npc.move_left()
Multiplayer Game Development
Introduction to Network Programming in Python
Use libraries like socket
for network programming to create multiplayer games.
Developing a Simple Multiplayer Game
Implement a basic client-server architecture where the server handles game logic, and clients communicate player actions.
Case Studies of Popular Python Games
Analysis of Successful Games Developed with Python
Analyze popular Python games like “EVE Online” and “Civilization IV” to understand best practices and successful strategies.
Lessons Learned and Best Practices from These Case Studies
- Prototyping: Rapid prototyping can help in refining game mechanics.
- Community Feedback: Engage with the community for valuable feedback and support.
Future Trends in Python Game Development
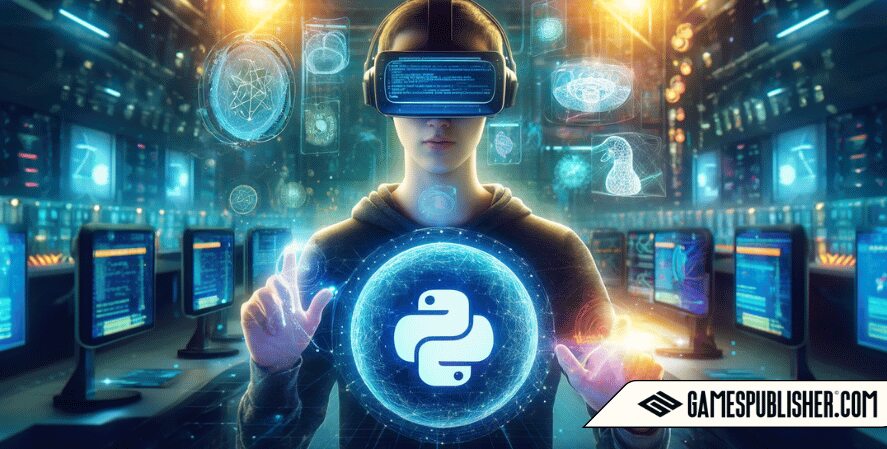
Emerging Technologies and Their Potential Impact on Python Games
Explore technologies like virtual reality (VR) and augmented reality (AR) and their integration with Python game development.
The Future of Python in the Game Development Industry
Python’s versatility and the continuous development of new libraries and tools ensure a bright future for Python in game development.
Conclusion
In this guide, we’ve covered the essentials of Python game development, from setting up your environment to creating and distributing your game. Python offers immense potential for game developers, thanks to its simplicity, readability, and extensive libraries.
Whether you’re a beginner or an experienced developer, Python provides the tools and community support needed to bring your game ideas to life. We encourage you to explore Python for your next game development project and join the thriving community of Python game developers.