In the dynamic and rapidly evolving world of game development, having access to the right resources can make all the difference. Gamespublisher.com stands as a premier resource for game developers and publishers, offering a wealth of insights, tools, and guidance tailored to the needs of the gaming industry.
Whether you’re a seasoned developer or just starting your journey, Gamespublisher.com is committed to providing valuable content that supports your growth and success in this exciting field.
Importance of JavaScript in Game Development
JavaScript has emerged as a powerful and versatile language in the realm of game development. Its simplicity and ease of use make it an ideal choice for beginners, while its robust capabilities cater to the needs of experienced developers.
JavaScript’s widespread adoption in web-based and mobile games highlights its flexibility and cross-platform potential. Understanding JavaScript not only enhances your game development skills but also opens up new opportunities in various gaming platforms and distribution channels.
Overview of JavaScript in Game Development
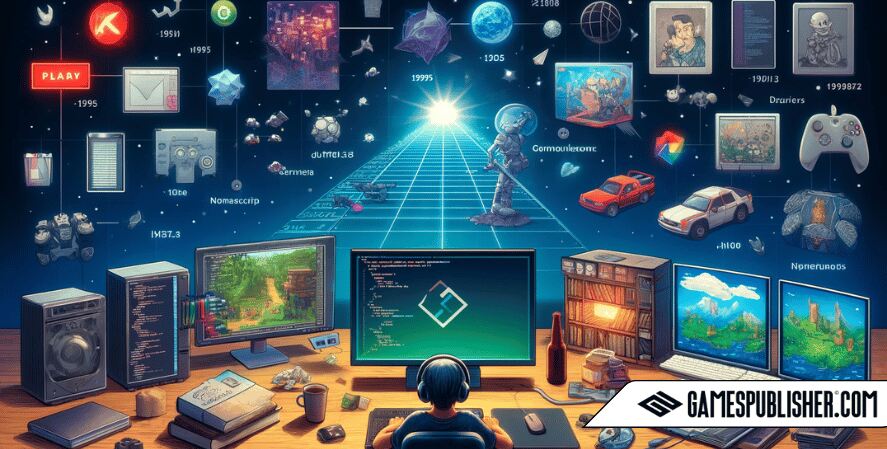
History and Evolution of JavaScript in Games
JavaScript was initially developed by Brendan Eich in 1995 as a scripting language for web browsers. Over the years, it has evolved significantly, transforming from a simple tool for adding interactivity to websites into a powerful programming language capable of creating complex applications, including games.
Key milestones in its evolution include the introduction of the ECMAScript standard, the development of powerful libraries and frameworks like Node.js, and the integration of HTML5 and CSS3, which have collectively expanded JavaScript’s capabilities in game development.
Why JavaScript for Game Development
JavaScript offers several advantages that make it a popular choice for game development:
- Simplicity: Its straightforward syntax and readability make it accessible for beginners.
- Community Support: A large and active community provides a wealth of resources, tutorials, and forums for troubleshooting and learning.
- Cross-Platform Capabilities: JavaScript can be used to develop games that run seamlessly on various platforms, including web browsers, mobile devices, and desktop applications.
- Integration with Web Technologies: JavaScript’s compatibility with HTML5 and CSS3 allows for the creation of rich, interactive game experiences.
Getting Started with JavaScript for Games
Setting Up the Development Environment
To start developing games with JavaScript, you’ll need to set up a suitable development environment. Here’s a step-by-step guide:
- Text Editor or IDE: Choose a code editor like Visual Studio Code, Sublime Text, or Atom.
- Web Browser: Use modern browsers like Google Chrome or Mozilla Firefox, which have excellent developer tools.
- Node.js: Install Node.js for running JavaScript outside the browser and managing dependencies.
- Version Control: Implement Git to monitor changes and facilitate collaboration with team members.
Basic Syntax and Concepts
Before diving into game development, it’s essential to understand the basic syntax and concepts of JavaScript:
Variables
javascriptCopy codelet playerName = 'Alice';
const maxScore = 100;
Data Types
javascriptCopy codelet isGameOver = false;
let playerScores = [10, 15, 20];
Functions
javascriptCopy codefunction startGame() {
console.log('Game started');
}
Control Structures
javascriptCopy codeif (playerScore >= maxScore) {
console.log('You win!');
}
Core JavaScript Techniques for Game Development
DOM Manipulation and Event Handling
JavaScript’s ability to interact with the Document Object Model (DOM) is crucial for game development. The DOM represents the structure of your web page, and you can manipulate it to create and control game elements.
Creating and Manipulating Elements
javascriptCopy codelet gameContainer = document.getElementById('gameContainer');
let newElement = document.createElement('div');
newElement.className = 'game-element';
gameContainer.appendChild(newElement);
Event Handling
javascriptCopy codedocument.addEventListener('keydown', function(event) {
if (event.key === 'ArrowUp') {
// Move player up
}
});
Canvas and Graphics
The HTML5 Canvas element is a powerful tool for rendering 2D graphics, essential for many types of games.
Setting Up the Canvas
htmlCopy code<canvas id="gameCanvas" width="800" height="600"></canvas>
javascriptCopy codelet canvas = document.getElementById('gameCanvas');
let ctx = canvas.getContext('2d');
Drawing Shapes and Images
javascriptCopy code// Draw a rectangle
ctx.fillStyle = 'blue';
ctx.fillRect(50, 50, 100, 100);
// Draw an image
let img = new Image();
img.src = 'path/to/image.png';
img.onload = function() {
ctx.drawImage(img, 200, 200);
};
Handling Animations
javascriptCopy codefunction draw() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
// Drawing code here
requestAnimationFrame(draw);
}
draw();
Game Loop and Timing
The game loop is the heartbeat of any game, continuously updating the game state and rendering frames.
Implementing a Game Loop
javascriptCopy codefunction gameLoop() {
update();
draw();
requestAnimationFrame(gameLoop);
}
gameLoop();
Timing Functions
Use setInterval
and requestAnimationFrame
for timing and animation control.
javascriptCopy codesetInterval(update, 1000 / 60); // Update 60 times per second
Advanced JavaScript Concepts for Game Development
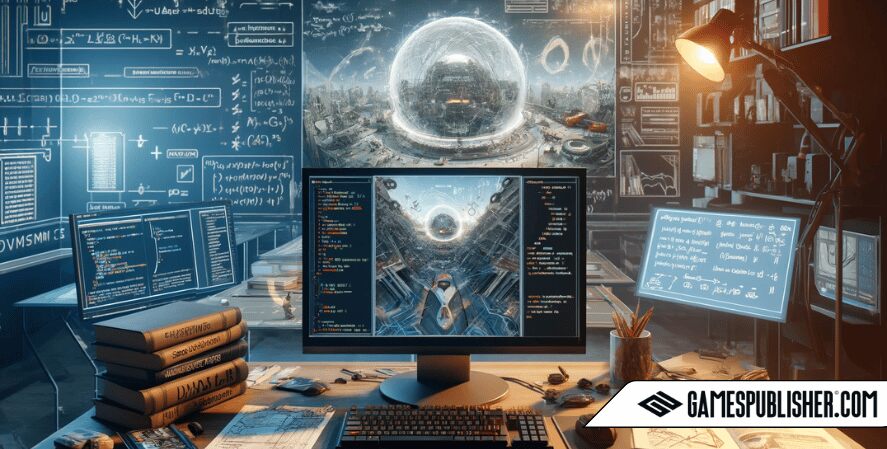
Object-Oriented Programming (OOP) in JavaScript
OOP principles help organize and structure your game code, making it more manageable and reusable.
Creating Game Objects
javascriptCopy codeclass Player {
constructor(name, score) {
this.name = name;
this.score = score;
}
updateScore(points) {
this.score += points;
}
}
let player1 = new Player('Alice', 0);
player1.updateScore(10);
Physics and Collision Detection
Implementing basic physics and collision detection adds realism to your games.
Bounding Box Collision
javascriptCopy codefunction isColliding(rect1, rect2) {
return rect1.x < rect2.x + rect2.width &&
rect1.x + rect1.width > rect2.x &&
rect1.y < rect2.y + rect2.height &&
rect1.y + rect1.height > rect2.y;
}
Handling User Input
Capturing user input is essential for interactive games.
Keyboard Input
javascriptCopy codedocument.addEventListener('keydown', function(event) {
switch(event.key) {
case 'ArrowUp':
// Move player up
break;
case 'ArrowDown':
// Move player down
break;
// Add more cases as needed
}
});
Mouse Input
javascriptCopy codecanvas.addEventListener('mousedown', function(event) {
let x = event.offsetX;
let y = event.offsetY;
// Handle mouse click at (x, y)
});
Integrating Libraries and Frameworks
Popular JavaScript Game Engines
Using game engines can significantly speed up development by providing pre-built functionalities.
Phaser
A fast, robust framework for 2D games.
javascriptCopy codeconst config = {
type: Phaser.AUTO,
width: 800,
height: 600,
scene: {
preload: preload,
create: create,
update: update
}
};
const game = new Phaser.Game(config);
function preload() {
this.load.image('sky', 'assets/sky.png');
}
function create() {
this.add.image(400, 300, 'sky');
}
function update() {
// Game logic here
}
Babylon.js
A powerful engine for 3D games.
javascriptCopy codeconst canvas = document.getElementById('renderCanvas');
const engine = new BABYLON.Engine(canvas, true);
const scene = new BABYLON.Scene(engine);
const camera = new BABYLON.ArcRotateCamera('camera', Math.PI / 2, Math.PI / 4, 4, BABYLON.Vector3.Zero(), scene);
camera.attachControl(canvas, true);
const light = new BABYLON.HemisphericLight('light', new BABYLON.Vector3(1, 1, 0), scene);
const sphere = BABYLON.MeshBuilder.CreateSphere('sphere', {}, scene);
engine.runRenderLoop(() => {
scene.render();
});
Using External Libraries
External libraries can enhance game functionality, such as adding physics or audio effects.
Matter.js for Physics
javascriptCopy codeconst { Engine, Render, World, Bodies } = Matter;
const engine = Engine.create();
const render = Render.create({
element: document.body,
engine: engine
});
const box = Bodies.rectangle(400, 200, 80, 80);
const ground = Bodies.rectangle(400, 610, 810, 60, { isStatic: true });
World.add(engine.world, [box, ground]);
Engine.run(engine);
Render.run(render);
Optimizing JavaScript Games
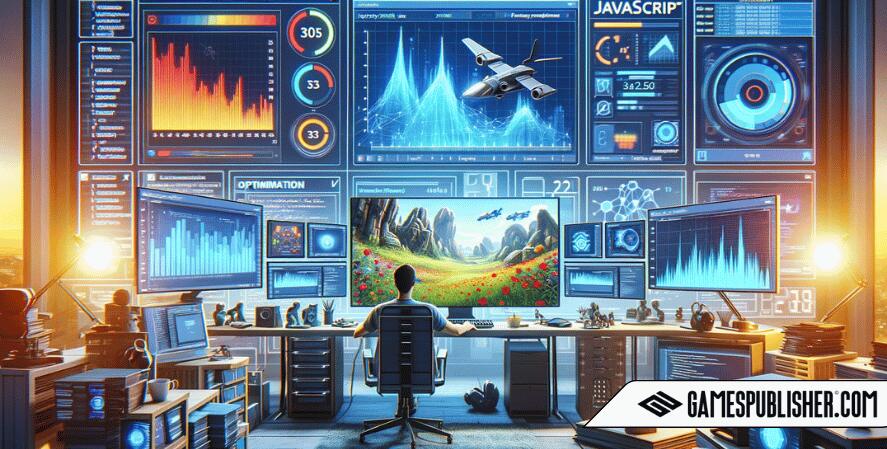
Performance Optimization Techniques
Optimizing your game ensures a smooth and responsive experience for players.
- Minimize DOM Manipulations: Batch updates and minimize reflows.
- Use Efficient Data Structures: Optimize algorithms and data storage.
- Optimize Rendering: Use techniques like sprite sheets and off-screen rendering.
Debugging and Testing
Robust debugging and testing practices are crucial for identifying and fixing issues.
Debugging Tools
Utilize browser developer tools to inspect and debug your code.
javascriptCopy codeconsole.log('Debug message');
Automated Testing
Write tests to ensure game functionality.
javascriptCopy code// Example using Jest
test('adds 1 + 2 to equal 3', () => {
expect(1 + 2).toBe(3);
});
Conclusion
Summary of Key Points
JavaScript is a versatile and powerful language for game development, offering simplicity, community support, and cross-platform capabilities. From basic syntax to advanced concepts, JavaScript provides the tools needed to create engaging and interactive games.
Encouragement to Experiment and Innovate
As you embark on your game development journey with JavaScript, remember that experimentation and innovation are key. Explore the vast resources available on Gamespublisher.com and beyond, and don’t be afraid to push the boundaries of what’s possible in your games.