Welcome to Gamespublisher.com, a reliable and helpful site for new game developers and publishers.
C# is a crucial programming language in today’s video game industry.
This programming language is extremely widely used by many game developers. Its simplicity, ease of learning, and effectiveness in handling complex games are the reason.
C# is extensively employed in popular game development tools like Unity, providing cross-platform capabilities such as documentation and useful tools.
Understanding C# can enhance game development skills and improve the quality and efficiency of game production. Therefore, video game publishers can craft intricate and distinctive games.
Overview of C# in Game Development
Let’s discover C#’s history and why C# is popular in game development!
History and Evolution of C#
Microsoft developed C# in the early 2000s.
Since then, it has gained global popularity and is used in many areas. Unity’s birth also widely boosted the use of C# in game programming.
The gaming world is always changing and competitive. Unity and C# have let independent and large companies make diverse and rich games.
Thus, C# has always adapted well to changes in this gaming industry. It keeps adding features and improving the dialect for amusement development.
Why C# is Popular in Game Development
C# is well known for its amusement advancement features.
The reason is that these features are easy to use and learn. Its development is versatile, ranging from 2D to VR, and integration with Unity is good.
Moreover, C# offers a wide range of libraries and excellent support for running on different operating systems.
Additionally, the community of C# is very large and active, providing many resources, including experience sharing and developers’ support.
Setting Up Your C# Development Environment
Here’s how to set up your C# development environment!
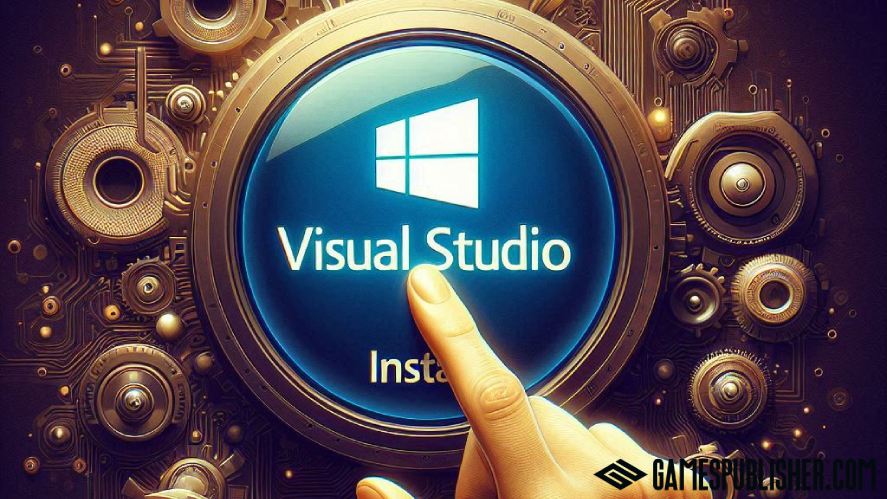
1. Installing Visual Studio
Visual Studio is one of the most popular Integrated Development Environments (IDEs) for the C# programming language.
Installing Visual Studio can be straightforward if you follow the steps below.
#1: Download Visual Studio
- Open your web browser and visit visualstudio.microsoft.com.
- Game publishers may choose free or paid options depending on their personal goals.
- Make your decision and click download.
#2: Run the Installer
- After downloading the installer, double-click on it to initiate the installation process.
- Visual Studio will take a few minutes to download the necessary components onto your computer.
#3: Choose Installation Components
- After the initial components are downloaded, the main installation window will appear.
- To start learning and developing applications using C#, choose “ASP.NET and web development” and “Desktop development with .NET” when setting up your development environment.
- You can select additional options if needed, but these two options are sufficient to get started.
#4: Install Visual Studio
- Presently, press the “Install” button in the right corner.
- The installation process may take some time, depending on your internet speed and computer configuration.
#5: Launch Visual Studio
- Once the installation process is finished, click the “Launch” button to start up Visual Studio.
- When you open VS, you need to sign in with your Microsoft account. You can also create a new account to sign in.
- After signing in, Visual Studio will ask you for initial preferences such as color theme and workspace settings. After you have selected your preferences, click on the “Start Visual Studio” button to begin.
#6: Create Your First C# Project
- After Visual Studio launches, you will see the welcome screen with options to create a new project or open an existing one.
- Click on “Create a new project”.
- In the new window, select “Console App (.NET Core)” or “Console App (.NET Framework)” depending on which version of .NET you want to use.
- Click “Next”.
- Name your projects and select a place to save them. Next, click the “Create” button.
- Visual Studio will extend the sample with fundamental source code. You can start writing your C# code and develop your application here.
2. Introduction to Unity
To start with Unity, a C# game engine, you must download and install the latest version from the official Unity website.
Once installed, you must create a free Unity account to access Unity’s features and services.
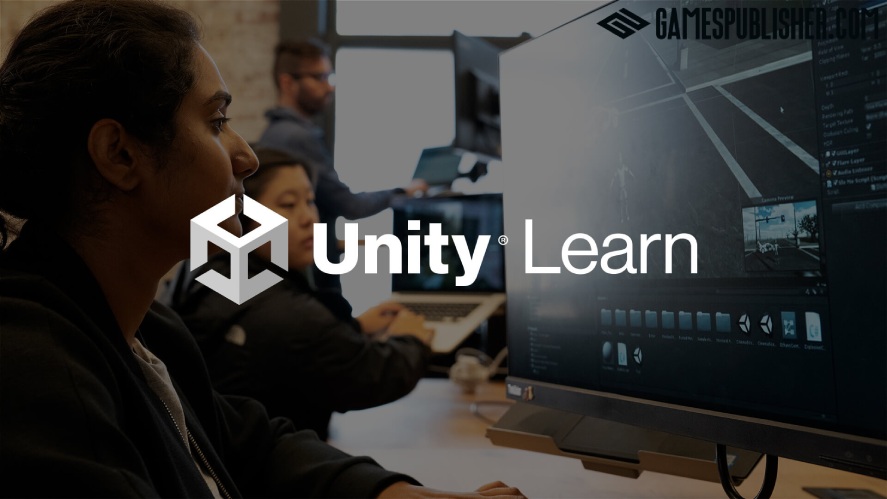
After that, you can create a new project. Everything from graphic design to code is provided in Unity.
3. Basic C# Syntax and Concepts
C# is used extensively in video game development, desktop applications, and web services. To start a programming journey of C#, you need to understand its basics.
So, the information below gives you an overview of C# syntax and some basic programming concepts.
1. Variables and Data Types
Variables are containers for storing data values. In C#, you must present a variable with a specific data type.
Common data types include:
- int: for integers (e.g., int age = 30;)
- double: for floating-point numbers (e.g., double price = 19.99;)
- char: for single characters (e.g., char grade = ‘A’;)
- string: for a series of characters (e.g., string name = “Kevin”;)
- bool: for boolean values (e.g., bool isStudent = true;)
2. Operators
Operators are characterized as symbols that perform operations on factors and values.
Common operators include:
- Arithmetic Operators: +, -, *, /, %
- Comparison Operators: ==, !=, >, <, >=, <=
- Logical Operators: &&, ||, !
3. Control Structures
Control structures allow you to control the flow of execution in your program.
The main control structures are:
- Conditional Statements: if, else if, else
- Loops: for, while, do-while
4. Methods
Blocks of code that perform a specific task are called methods.
Methods are defined within a class and can be called to execute when needed.
5. Classes and Objects
C# is an object-oriented dialect, which implies it employs classes and objects to model real-world substances.
A class is a blueprint for generating objects and defining their attributes and behaviors.
Creating Your First Game in C#
This section covers planning your game, writing your first C# script in Unity, and implementing basic game mechanics.
Planning Your Game
When developing your game, designing and planning before coding is crucial.
The game design plan document is the first thing you must do. It will detail components such as gameplay, illustrations, and sound.
This document also helps the development team. All members of your team will understand the project’s goals and direction very clearly.
Furthermore, basic project planning involves dividing tasks and setting timelines for each part. This guarantees a seamless project flow and eliminates risks and errors.
Writing Your Script
When writing your first script in Unity, you start by opening Unity and creating a new C# script.
Title the script and open it in your code editor. Inside, you’ll see two main functions:
- Start(): runs once when the game starts.
- Update(): runs every frame.
You can write simple code in these functions to make things happen in your game.
For example, add code to move an object in the Update() function.
Implementing Game Mechanics
To implement basic game mechanics, start with player movement.
Use simple code to make the player move left, right, up, and down. Next, add scoring by increasing the score when the player collects items or achieves goals.
You can display the score on the screen to keep the player informed.
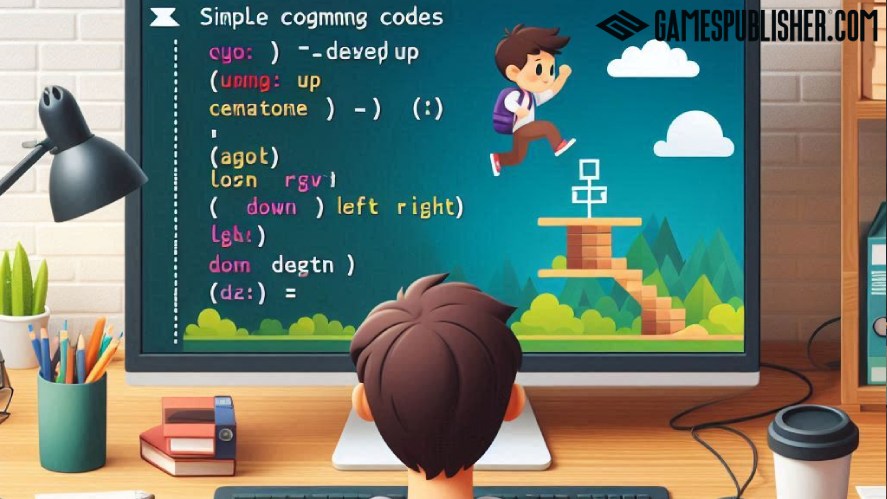
After that, manage the game state by setting up conditions for starting, pausing, and ending the game.
For example, the game can start when the player presses a button and pause when another button is pressed.
Finally, ensure the game over condition is clear, such as when the player loses all lives.
This helps keep the game organized and ensures everything works smoothly, making the gameplay experience enjoyable.
Advanced C# Programming Techniques for Games
Let’s discover advanced C# programming techniques tailored for game development!
Object-Oriented Programming (OOP) in C#
Object-Oriented Programming (OOP) in C# is important for game development.
It involves concepts such as:
- Classes: These are blueprints for objects.
- Objects: Instances of classes.
- Inheritance: Where one class can use methods and properties of another.
- Encapsulation: Hides an object’s inner workings, letting only specific functions access them.
- Polymorphism: Where objects can act like instances of their parent classes.
Overall, OOP helps organize game code, making it reusable and simpler.
Data Structures, Algorithms, and Performance Optimization
In game development, important tools are data structures and algorithms. These help manage and process data efficiently.
For example, trees and graphs organize data hierarchically and in networks. Moreover, pathfinding algorithms find the best routes for characters or objects in games.
Performance optimization in C# is crucial for better game performance.
Tips include using efficient algorithms, minimizing memory usage, and reducing unnecessary computations.
Managing resources well and leveraging hardware capabilities also improve game speed and smoothness.
Debugging and Testing C# Games
Debugging and testing C# Games are crucial for game development, ensuring smoother gameplay and fewer technical issues.
Common Bugs in C# Game Development
In C# improvement, developers regularly confront common bugs and issues.
To begin with, invalid reference blunders happen when the code tries to utilize an object that has not been initialized.
Following memory spills happen when the program doesn’t discharge unused memory, causing it to moderate or crash.
Additionally, logic errors, where the code doesn’t work as intended, can be tricky to find and fix.
Furthermore, performance issues arise when the game runs slowly due to inefficient code.
Finally, compatibility problems can occur when the game doesn’t work well on different devices or operating systems.
These bugs are frustrating, but understanding them helps developers find solutions more quickly.
Debugging Tools and Techniques
For instance, you can utilize breakpoints to halt the code and check the values of factors.
You can also step through the code line by line to get its flow. The console window is another useful tool for displaying error messages and logs.
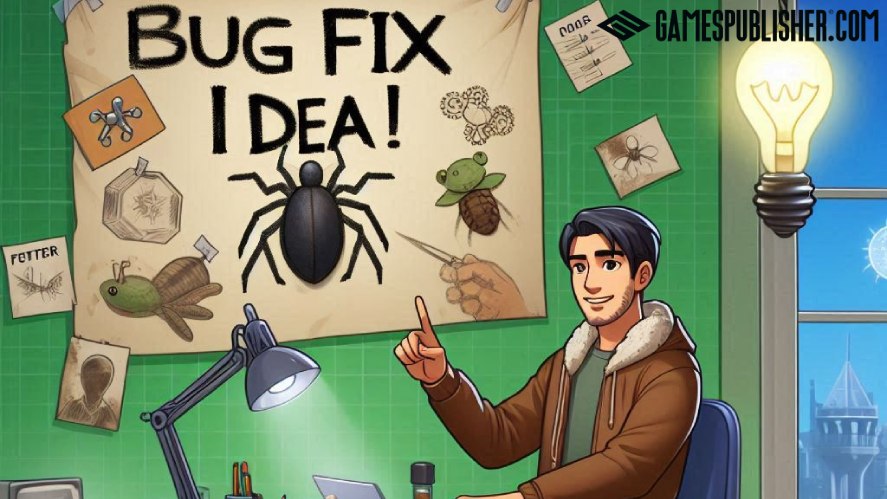
Moreover, ensuring your code is clear and straightforward enhances the ease of debugging. Developers must regularly test and identify them to help the game get bugs fixed early.
Using these tools and techniques makes debugging more efficient and effective.
Automated Testing
Automated testing is very important because it helps ensure the quality and reliability of the game. It involves creating tests that run automatically to check if different parts of the game work as expected.
In C#, implementing unit tests is a common practice where small code units are tested individually.
The result is that developers can find bugs early and ensure changes don’t break existing features.
Publishing Your C# Game
Let’s focus on the steps involved in releasing your C# game!
Preparing for Release
Preparing a game for release involves several important steps.
First, conduct final testing to ensure the game is free of bugs and runs smoothly.
Test on different devices and operating systems to catch any issues.
Next, graphics, sound, and interface also need to be improved. This will make the game more appealing and ensure it is balanced and fun.
After that, package the game by creating installers or uploading it to app stores. Write clear instructions and descriptions for players to download and enjoy.
Publishing Gaming Platforms and Marketing Your Game
There are many video game distribution platforms where you can publish your C# games.
One popular platform is Steam. This large community of gamers is great for reaching many players.
Another choice is itch.io, which gives indie developers much freedom in selling their games.
The Microsoft Store is also a good choice if you are targeting Windows users. It is easy to integrate with Windows features.
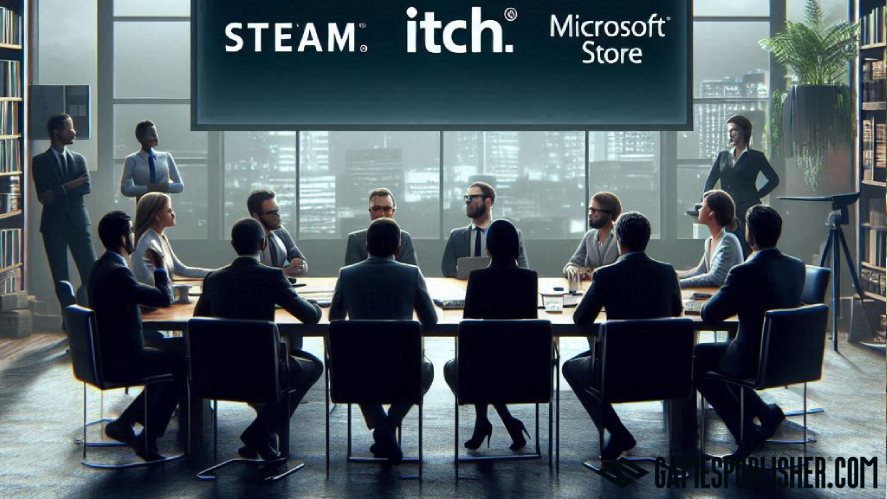
Each platform has its benefits. So, developers should choose the one that best fits each game and its audience.
To effectively advertise your game with your target audience, learn where they spend time online and get your players.
Engage them through social media and gaming communities, and explore collaborations with influencers to boost your game’s visibility.
Conclusion
That are the key aspects of developing games with C#, with basic programming concepts, and a practical guide for beginners.
Developers should continue learning and enhancing skills in C# game development. Also, practice coding regularly, try different game mechanics, and get inspiration from existing games to improve.
Remember to check the website Gamespublisher.com for more resources and tutorials on game development.